When you create an email, letter or phone call through SDK (Plugin, Workflow or from your website or Silverlight application) you might get the error 'Creating Entity with an invalid parent. Entity: Email'
The reason behind this is your regarding object does not support activity association.
All you have to do is navigate the entity from Customisation and tick Activities in the 'Communication & Collaboration' section and publish the entity.
Hope this will help.
Microsoft Dynamic CRM
Friday, December 13, 2013
Tuesday, May 28, 2013
Moving the notes section to the top of the page
When you move the notes section to the top of the form and when the form is loaded, it automatically scrolls down and user has to manually scroll up to access the notes section.
If you want to see the notes section without scrolling please proceed the below steps.
1. If your have already added JQuery web resource to the form then just copy below code to a function and call at form- onload
2. Or if you prefer the javascrips then just copy below code to a function and call at form- onload
If you want to see the notes section without scrolling please proceed the below steps.
1. If your have already added JQuery web resource to the form then just copy below code to a function and call at form- onload
$("#crmFormTabContainer").scrollTop(0);
2. Or if you prefer the javascrips then just copy below code to a function and call at form- onload
document.getElementById('crmFormTabContainer').scrollTop = 0;
Friday, February 22, 2013
Get System Users From Specific Team
Today I had a very simple request, which is to get System Users in a specific team. Since it was a very simple task I just Google and try to find a sample. I was amazed as there was no such exaample in the Net. So I had to write my own. Once it is done, I just thought to share it as it might be useful.
Here is the code.
1. First example is to get the System Users in a Team
2. Second example is to get System Users as ActivityParty that you might need to link with an Activity.
Here is the code.
1. First example is to get the System Users in a Team
2. Second example is to get System Users as ActivityParty that you might need to link with an Activity.
public List<SystemUser> GetTeamUsers(string teamName) { return (from user in Context.CreateQuery<SystemUser>() join teamMembership in Context.CreateQuery<TeamMembership>() on user.SystemUserId.Value equals teamMembership.SystemUserId join team in Context.CreateQuery<Team>() on teamMembership.TeamId.Value equals team.TeamId.Value where team.Name == teamName select user ).ToList(); }
public List<ActivityParty> GetTeamUsersAsActivityParty(string teamName) { return (from user in Context.CreateQuery<SystemUser>() join teamMembership in Context.CreateQuery<TeamMembership>() on user.SystemUserId.Value equals teamMembership.SystemUserId join team in Context.CreateQuery<Team>() on teamMembership.TeamId.Value equals team.TeamId.Value where team.Name == teamName select new ActivityParty { PartyId = new EntityReference { LogicalName = user.LogicalName, Id = user.SystemUserId.Value } }).ToList(); }
Thursday, September 6, 2012
How to join 2 entities in LINQ when where clause is not constant
When we join 2 entities using linq in C#, in where clause has fixed variables in most of the times.
For an example, we want to find records where the Lookup Id is equal to a constant value or where clause is greater than for a specific date.
In this kind of cases, we can write very simple linq query.
But sometimes we have to join 2 (or more) entities and we want to filter records with the variables in between them.
Example
We have 1 to many relationship with contacts and visits
And we want to get contact Ids where Contact Agreement Date is greater than Visit Date. And hence Contact.AgreementDate and Visit.VisitDate are not constant.
We can write the query in SQL as below
If we write this in C#, we get below query
Check the right hand side of the below where clase
But this can be done using anonymous types.
For an example, we want to find records where the Lookup Id is equal to a constant value or where clause is greater than for a specific date.
In this kind of cases, we can write very simple linq query.
But sometimes we have to join 2 (or more) entities and we want to filter records with the variables in between them.
Example
We have 1 to many relationship with contacts and visits
And we want to get contact Ids where Contact Agreement Date is greater than Visit Date. And hence Contact.AgreementDate and Visit.VisitDate are not constant.
We can write the query in SQL as below
select contact.Id from new_visit visit inner join Contact on visit.new_contactId = contact.ContactId
and visit.new_VisitDate >= contact.new_AgreementDate where visit.statecode = 0 and contact.StateCode = 0
If we write this in C#, we get below query
var query = from visit in context.CreateQuery<new_visit>() join contact in context.CreateQuery<Contact>() on visit.new_contactId.Id equals contact.ContactId where visit.new_VisitDate >= contact.new_AgreementDate where visit.statecode.Value == new_visitState.Active where contact.statecode.Value == ContactState.Active select contact.Id
Check the right hand side of the below where clase
where visit.new_VisitDate >= contact.new_AgreementDatesince the right side is not constant, Linq cannot query the data we want.
But this can be done using anonymous types.
IOrganizationService service = new CRMInternal().GetService(); OrganizationServiceContext context = new OrganizationServiceContext(service); var query = from visit in context.CreateQuery<new_visit>() join contact in context.CreateQuery<Contact>() on visit.new_contactId.Id equals contact.ContactId where visit.statecode.Value == new_visitState.Active where contact.StateCode.Value == ContactState.Active select new { contact.Id, visit.new_VisitDate, contact.new_AgreementDate }; var contacts = query.ToList().Where(p => p.new_VisitDate <= p.new_AgreementDate).Select(p => p.Id);
Thursday, July 26, 2012
Metadata contains a reference that cannot be resolved: 'http://Server:port/Org/XRMServices/2011/Organization.svc?wsdl'.
I've been connecting my CRM Development Server for last few months and today I installed CRM Outlook Client to my Local PC.
Once I installed the CRM Outlook Client, I couldn't connect to CRM.
My PC is 64 bit and I have 32 bit MS Office(and Outlook). I think that might be a reason that the CRM Outlook Client did not work. ( I got some errors when installing but was manged to install by manually installing "Microsoft Online Services Sign-in Assistance")
Since it did not work properly, I uninstalled the CRM Outlook Client. But just after that, and start my usual work I got following error
Metadata contains a reference that cannot be resolved: 'http://Server:port/Org/XRMServices/2011/Organization.svc?wsdl'.
After doing some research, I found that my machine.config file has been modified today.
I compared the "machine.config" file with one of colleague's PC and found the additional tag.
<system.net>
<defaultproxy enabled="true" usedefaultcredentials="true">
<proxy bypassonlocal="True" proxyaddress="###.com.sg">
</proxy>
</defaultproxy>
</system.net>
I then removed the whole "<system.net>" tag from the "machine.config" file. Everything is working fine now!
If you found the same error, remember to get a backup of the "machine.config" file before proceeding!
Since it did not work properly, I uninstalled the CRM Outlook Client. But just after that, and start my usual work I got following error
Metadata contains a reference that cannot be resolved: 'http://Server:port/Org/XRMServices/2011/Organization.svc?wsdl'.
After doing some research, I found that my machine.config file has been modified today.
I compared the "machine.config" file with one of colleague's PC and found the additional tag.
<system.net>
<defaultproxy enabled="true" usedefaultcredentials="true">
<proxy bypassonlocal="True" proxyaddress="###.com.sg">
</proxy>
</defaultproxy>
</system.net>
I then removed the whole "<system.net>" tag from the "machine.config" file. Everything is working fine now!
If you found the same error, remember to get a backup of the "machine.config" file before proceeding!
Tuesday, July 17, 2012
CRM Developers have more time to learn cross browser supporting Javascripts!
Microsoft earlier announced that they will include cross-browser support with CRM 2011 - Q2 2012 Service Update, and what that means is end-users will be able to login to CRM using browsers like Internet Explorer, Chrome, Firefox and Safari. Users can login to CRM 2011 from their mobile devices such as iPad, iPhone, Android, Blackberry and Windows Phone devices.
In most of CRM systems, we see developers include custom web pages to extend basic CRM features. We should try to minimize using custom web pages, and we should only implement such if the in-built Process Dialogs cannot full fill the customer requirements. We see Microsoft adding more features to Process Dialogs in rollups and sometimes we face customers, who really need some web pages like typical web pages! That’s when we have to implement custom web pages that we embedded to CRM Ribbon menu or CRM pages through IFrames. As CRM developers, we always checked our custom web page in Internet Explorer and if it works fine in IE, we just stop testing as we know uses cannot navigate to our pages using other browsers.
Microsoft just recently communicated that they are not including cross browser feature in the Q2 Service Update! They are planning to include the feature with the Q4 release. What is means to CRM developers? We have some more time to learn cross browser supporting Javascripts in our customer web pages. Because, once the end user start the CRM application from browsers not internet explorer browsers, we have to make sure our custom web pages also displays the content correctly and Javascripts work correctly.
So let’s utilize the additional time we got to learn more Javascripts and HTML that supports cross browser. And let’s make sure any web page we implement in future supports cross browser.
Some useful resources,
Microsoft delays Dynamics CRM mobile support for iPad, Android until Q4 2012
Q2 2012 Service Update – New delivery schedule
In most of CRM systems, we see developers include custom web pages to extend basic CRM features. We should try to minimize using custom web pages, and we should only implement such if the in-built Process Dialogs cannot full fill the customer requirements. We see Microsoft adding more features to Process Dialogs in rollups and sometimes we face customers, who really need some web pages like typical web pages! That’s when we have to implement custom web pages that we embedded to CRM Ribbon menu or CRM pages through IFrames. As CRM developers, we always checked our custom web page in Internet Explorer and if it works fine in IE, we just stop testing as we know uses cannot navigate to our pages using other browsers.
Microsoft just recently communicated that they are not including cross browser feature in the Q2 Service Update! They are planning to include the feature with the Q4 release. What is means to CRM developers? We have some more time to learn cross browser supporting Javascripts in our customer web pages. Because, once the end user start the CRM application from browsers not internet explorer browsers, we have to make sure our custom web pages also displays the content correctly and Javascripts work correctly.
So let’s utilize the additional time we got to learn more Javascripts and HTML that supports cross browser. And let’s make sure any web page we implement in future supports cross browser.
Some useful resources,
Microsoft delays Dynamics CRM mobile support for iPad, Android until Q4 2012
Q2 2012 Service Update – New delivery schedule
Tuesday, April 3, 2012
Dynamically changing Label Size in CRM fields
In the most of the times, we can manage the default width CRM provides to show a valid text to CRM labels. But there are occasions , that the customers really asking to put a lengthily text in to a CRM and they want it in one Line!
By default this cannot be possible, but still this can be implement by over writing CRM Table Styles in the Specific table.
For this you can do below steps.
1.create sections for each field, so the over write behavior will not effect to other fields in the Page.
2. Call the Functions for each and every field.
Example
Function: SetLabelWidth
Parameters: 'new_age', 144
3. Copy the SetLabelWidth function to the JavaScript Library
By default this cannot be possible, but still this can be implement by over writing CRM Table Styles in the Specific table.
For this you can do below steps.
1.create sections for each field, so the over write behavior will not effect to other fields in the Page.
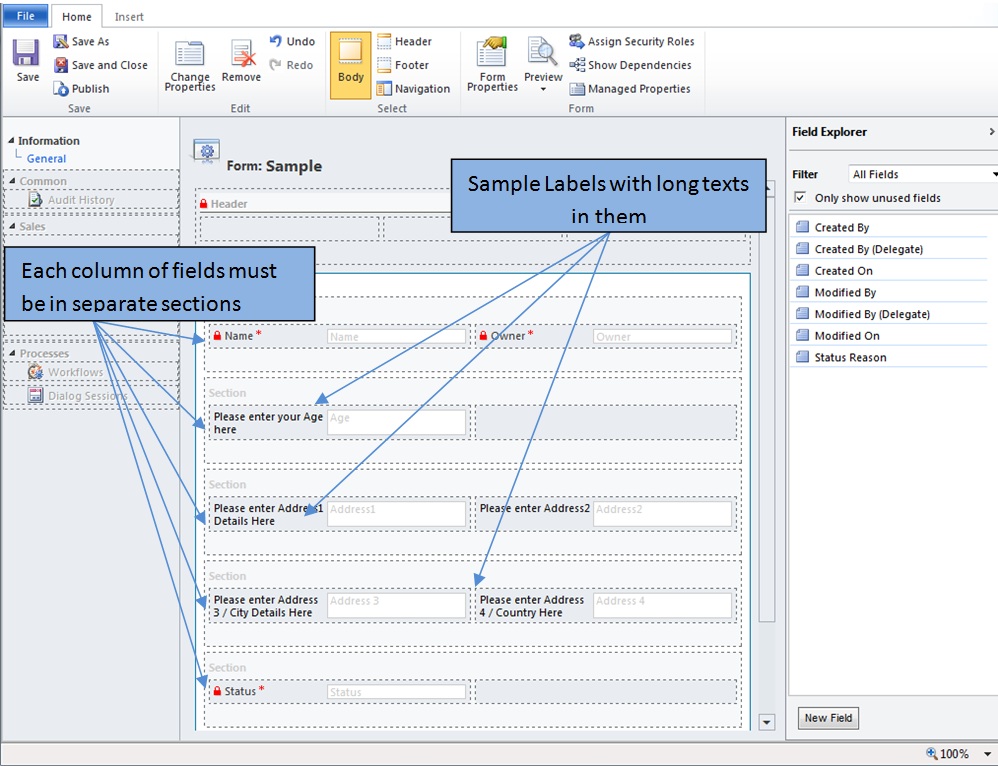
2. Call the Functions for each and every field.
Example
Function: SetLabelWidth
Parameters: 'new_age', 144
3. Copy the SetLabelWidth function to the JavaScript Library
function SetLabelWidth(targetLabelName, newWidth) { var targetTable = null; var colIndex = 0; var sectionId; var tabs = Xrm.Page.ui.tabs; for (var i = 0; i < tabs.getLength(); i++) { var tab = tabs.get(i); var sections = tab.sections; for (var j = 0; j < sections.getLength(); j++) { var section = sections.get(j); var controls = section.controls; for(var k = 0; k < controls.getLength(); k++) { if(controls.get(k).getName() == targetLabelName) { sectionId = section._control._element.id; colIndex = k + 1; } } } } targetTable = document.getElementById(sectionId); if(targetTable == null) { //Request field not found in any of the tables return; } var continerTableWidth = targetTable.clientWidth; var td1W = targetTable.rows[0].cells[0].clientWidth; var td2W = targetTable.rows[0].cells[1].clientWidth; var td3W = targetTable.rows[0].cells[2].clientWidth; var td2C = targetTable.rows[0].cells[1].all[0].offsetWidth; var td4W = 0; var td4C = 0; var sectionPadding = 20 var cellPading = 3; if(targetTable.rows[0].cells[3] != null) { td4W = targetTable.rows[0].cells[3].clientWidth; td4C = targetTable.rows[0].cells[3].all[0].offsetWidth; } else { td3W = continerTableWidth / 2; } //Break the Table Style targetTable.style.tableLayout = ""; targetTable.width = continerTableWidth; var controlNewWidth = 0; if(colIndex == 1) { controlNewWidth = td1W + td2W - newWidth; targetTable.rows[0].cells[0].width = newWidth; targetTable.rows[0].cells[1].width = controlNewWidth; if(td4W == 0) { targetTable.rows[0].cells[2].width = td3W; } else { targetTable.rows[0].cells[2].width = td3W - sectionPadding; targetTable.rows[0].cells[3].width = td4W - (cellPading * 2); targetTable.rows[0].cells[3].all[0].style.width = td4C; } } else if(colIndex == 2) { controlNewWidth = td3W + td4W - newWidth; targetTable.rows[0].cells[0].width = td1W + (cellPading * 2); targetTable.rows[0].cells[1].width = td2W; targetTable.rows[0].cells[2].width = newWidth - sectionPadding; targetTable.rows[0].cells[3].width = controlNewWidth; targetTable.rows[0].cells[1].all[0].style.width = td2C; targetTable.rows[0].cells[3].all[0].style.width = controlNewWidth - (cellPading * 2); } else { return; } }Then the fields will be display as below
Subscribe to:
Posts (Atom)