By default this cannot be possible, but still this can be implement by over writing CRM Table Styles in the Specific table.
For this you can do below steps.
1.create sections for each field, so the over write behavior will not effect to other fields in the Page.
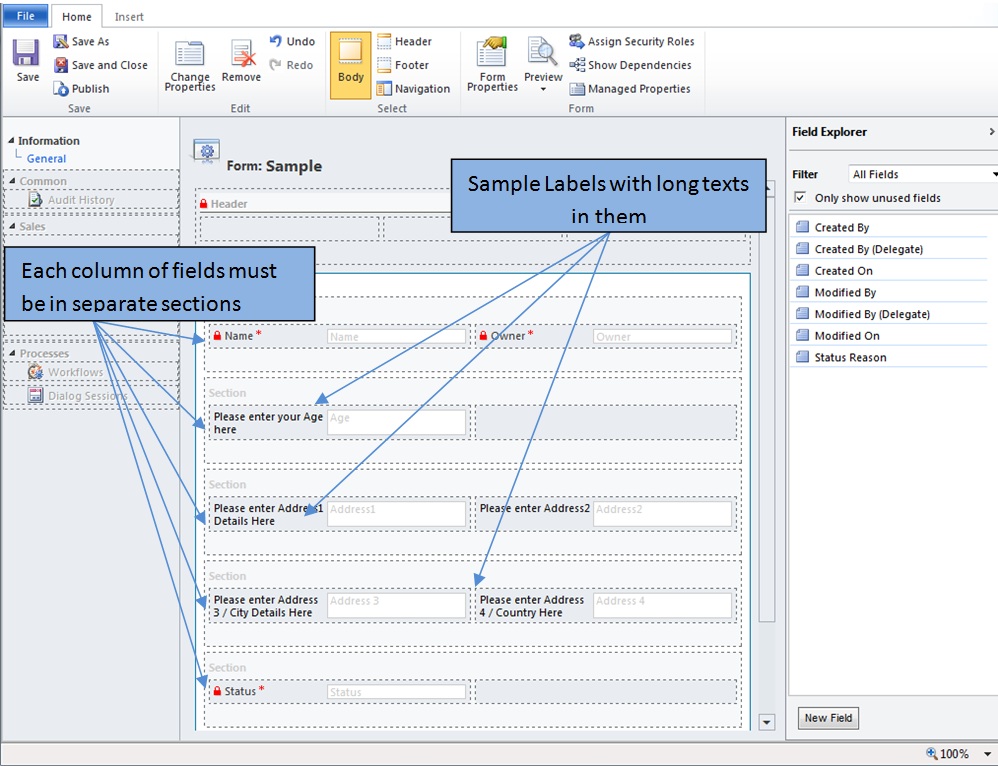
2. Call the Functions for each and every field.
Example
Function: SetLabelWidth
Parameters: 'new_age', 144
3. Copy the SetLabelWidth function to the JavaScript Library
function SetLabelWidth(targetLabelName, newWidth) { var targetTable = null; var colIndex = 0; var sectionId; var tabs = Xrm.Page.ui.tabs; for (var i = 0; i < tabs.getLength(); i++) { var tab = tabs.get(i); var sections = tab.sections; for (var j = 0; j < sections.getLength(); j++) { var section = sections.get(j); var controls = section.controls; for(var k = 0; k < controls.getLength(); k++) { if(controls.get(k).getName() == targetLabelName) { sectionId = section._control._element.id; colIndex = k + 1; } } } } targetTable = document.getElementById(sectionId); if(targetTable == null) { //Request field not found in any of the tables return; } var continerTableWidth = targetTable.clientWidth; var td1W = targetTable.rows[0].cells[0].clientWidth; var td2W = targetTable.rows[0].cells[1].clientWidth; var td3W = targetTable.rows[0].cells[2].clientWidth; var td2C = targetTable.rows[0].cells[1].all[0].offsetWidth; var td4W = 0; var td4C = 0; var sectionPadding = 20 var cellPading = 3; if(targetTable.rows[0].cells[3] != null) { td4W = targetTable.rows[0].cells[3].clientWidth; td4C = targetTable.rows[0].cells[3].all[0].offsetWidth; } else { td3W = continerTableWidth / 2; } //Break the Table Style targetTable.style.tableLayout = ""; targetTable.width = continerTableWidth; var controlNewWidth = 0; if(colIndex == 1) { controlNewWidth = td1W + td2W - newWidth; targetTable.rows[0].cells[0].width = newWidth; targetTable.rows[0].cells[1].width = controlNewWidth; if(td4W == 0) { targetTable.rows[0].cells[2].width = td3W; } else { targetTable.rows[0].cells[2].width = td3W - sectionPadding; targetTable.rows[0].cells[3].width = td4W - (cellPading * 2); targetTable.rows[0].cells[3].all[0].style.width = td4C; } } else if(colIndex == 2) { controlNewWidth = td3W + td4W - newWidth; targetTable.rows[0].cells[0].width = td1W + (cellPading * 2); targetTable.rows[0].cells[1].width = td2W; targetTable.rows[0].cells[2].width = newWidth - sectionPadding; targetTable.rows[0].cells[3].width = controlNewWidth; targetTable.rows[0].cells[1].all[0].style.width = td2C; targetTable.rows[0].cells[3].all[0].style.width = controlNewWidth - (cellPading * 2); } else { return; } }Then the fields will be display as below